If you’re working with TestNG, you’ve probably heard about listeners. These are tools that can step in during your test runs and tweak the default behavior. Simply put, TestNG Listeners let you decide what to do when a test starts, passes, fails, or gets skipped. Think of it like having a backstage crew for your tests. They’re super handy for creating detailed reports or logs tailored to your needs. Once you get the hang of it, listeners can really level up your test automation game.
Table of Contents
Types of TestNG Listeners
TestNG has several types of listeners, each with its own unique purpose. Here’s a quick rundown:
ITestListener
: Keeps tabs on individual test methods—great for tracking when tests pass, fail, or skip.ISuiteListener
: Monitors the start and end of a test suite.IReporter
: Helps create custom reports after your tests run.IAnnotationTransformer
: Lets you modify annotations dynamically at runtime.IInvokedMethodListener
: Watches over method-level events.IHookable
: Gives you control over the execution of methods.IConfigurationListener
: Tracks the execution of configuration methods.IMethodInterceptor
: Adjusts the order in which your test methods run.
Each of these is useful depending on what you’re trying to achieve. For example, ITestListener
is often used to log test outcomes or capture screenshots on failure, making debugging easier. ISuiteListener
is ideal for monitoring the lifecycle of a suite, such as setting up global resources before tests start or cleaning them up afterward. If you’re creating custom reports, IReporter
is the go-to option, while IAnnotationTransformer
can help dynamically adjust test parameters at runtime. By picking the right listener, you can make your testing process more efficient and tailored to your specific needs.
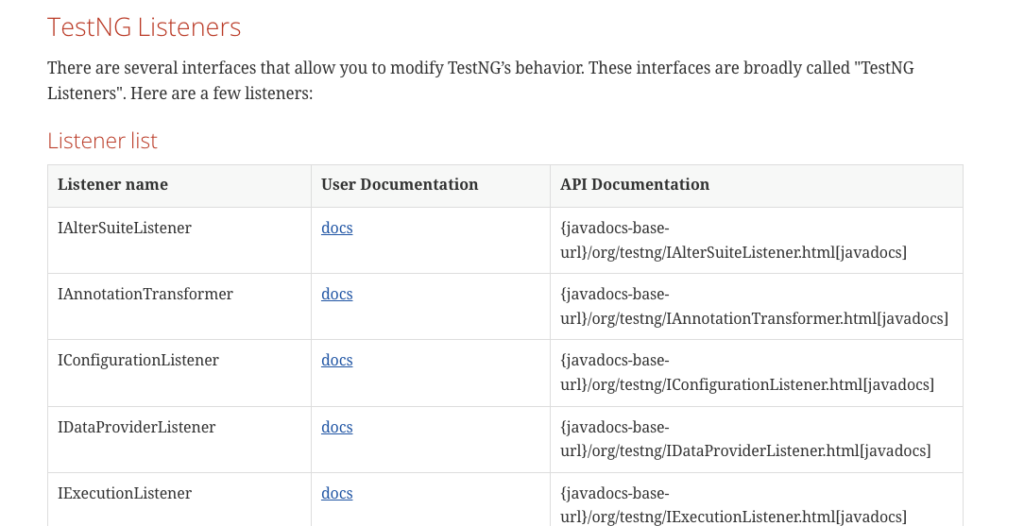
Implementing ITestListener
Interface
The ITestListener
interface is one of the most commonly used listeners. Let’s break it down step-by-step:
Step 1: Create a Class
Start by making a new class that implements ITestListener
:
import org.testng.ITestListener;
import org.testng.ITestResult;
public class CustomTestListener implements ITestListener {
@Override
public void onTestStart(ITestResult result) {
System.out.println("Test started: " + result.getName());
}
@Override
public void onTestSuccess(ITestResult result) {
System.out.println("Test passed: " + result.getName());
}
@Override
public void onTestFailure(ITestResult result) {
System.out.println("Test failed: " + result.getName());
}
@Override
public void onTestSkipped(ITestResult result) {
System.out.println("Test skipped: " + result.getName());
}
}
Step 2: What Do These Methods Do?
onTestStart()
: Runs when a test kicks off.onTestSuccess()
: Fires when a test passes.onTestFailure()
: Perfect for adding actions like taking screenshots.onTestSkipped()
: Executes if a test gets skipped.
Step 3: Practical Use
Want better logs or email notifications? You can enhance your logs by integrating a logging library like Log4j or SLF4J to capture detailed test execution events. For email notifications, you could use a tool like JavaMail API to send test results or alerts directly to your inbox. For example, you might configure the onTestFailure()
method to log a detailed error message and simultaneously trigger an email notification with a link to the failure logs or screenshots.
Using @Listeners
Annotation
One of the easiest ways to use a listener is with the @Listeners
annotation. This method is preferred by many developers because it simplifies the process of integrating listeners into specific test classes, making it easier to customize logging or reporting for individual test cases without affecting the global configuration. Here’s how you do it:
How to Add @Listeners
import org.testng.annotations.Listeners;
@Listeners(CustomTestListener.class)
public class TestClass {
@Test
public void sampleTest() {
Assert.assertTrue(true);
}
}
Why Use @Listeners
?
- It’s a simple way to attach listeners to specific classes.
- You can focus on particular tests without making global changes.
Example Output
When you run this, the messages from CustomTestListener
will show up in your logs, reflecting each event the listener tracks, like test starts, passes, failures, or skips. These outputs confirm that the listener is working as expected and can be a great way to debug or verify the flow of your test cases.
Configuring Listeners in testng.xml
If you want to apply listeners to all your tests, the testng.xml
file is the way to go.
Adding Listeners in testng.xml
<suite name="Test Suite">
<listeners>
<listener class-name="CustomTestListener" />
</listeners>
<test name="Test">
<classes>
<class name="TestClass" />
</classes>
</test>
</suite>
Why Use This?
- It’s great for large projects where consistent logging or reporting is key because it ensures that all test cases follow the same standards, making it easier to debug and analyze results. For example, you can use it to automatically log test outcomes to a central dashboard or generate uniform reports for the entire test suite.
Real Example
For big test suites, this centralized approach ensures that everything stays organized and follows the same standards. Developers often use this method to set up global logging frameworks or manage reporting tools that track results across the entire suite. For example, you can define a listener in testng.xml
to log each test’s execution and capture errors for deeper analysis, making debugging smoother and more efficient.
Practical Applications of TestNG Listeners
TestNG listeners are like Swiss Army knives—they’re good for so many things. For instance, you could use a listener to automatically log when a test starts or to capture a screenshot the moment a test fails. Imagine a scenario where you’re debugging a flaky test: the listener can record every detail about the test’s execution and pinpoint exactly where it went wrong, saving you hours of manual analysis. Here are some examples:
Take Screenshots on Failure
@Override
public void onTestFailure(ITestResult result) {
takeScreenshot(result.getName());
}
Custom Logging
Use a logger like Log4j to create detailed execution logs.
Generate Custom Reports
Use the IReporter
interface to make reports that look exactly how you want.
Real-World Scenario
Combine ITestListener
and IReporter
for advanced logging and custom reports tailored to your team’s needs.
Best Practices for Using TestNG Listeners
Keep It Simple
- Don’t overload listeners with heavy logic.
- Stick to essential tasks like logging and reporting.
Watch Out for Pitfalls
- Using too many listeners at once can make things messy.
- Always test your listeners to avoid surprises during test runs.
Be Mindful of Performance
To keep your tests running smoothly, avoid adding heavy tasks in listener methods. For example, instead of writing large logs directly to a file, use asynchronous logging tools like Log4j to offload tasks efficiently. Similarly, if you need to trigger email notifications, queue them for processing after the test run rather than doing it in real-time.
Conclusion
TestNG listeners are a game-changer when it comes to customizing your test automation. They’re perfect for capturing screenshots, logging details, and generating custom reports. Start small by implementing ITestListener
, and as you get comfortable, explore other types of listeners. They’re easy to integrate and can make your test framework way more powerful. Give them a shot—you’ll wonder how you ever managed without them!
FAQs
What is the use of listeners in TestNG?
Listeners in TestNG allow you to customize the behavior of your tests based on specific events. They can modify test execution, generate custom reports, and provide real-time feedback.
What is an example of a listener in TestNG?
A common example is the ITestListener
, which provides methods like onTestStart
and onTestFailure
to perform actions at different stages of test execution.
What is the use of @listener annotation?
The @Listeners
annotation in TestNG is used to specify which listener class should be associated with a particular test class, allowing for custom behavior during test execution.
What is the use of a listener in general?
In the broader context, a listener is a design pattern that allows an object to “listen” to specific events or changes in another object. It provides a mechanism for one object to notify other objects of any state changes, ensuring a dynamic and responsive system.