TestNG is a powerful Java testing framework inspired by JUnit. It offers advanced features, especially for integration testing. One standout feature is the DataProvider, a vital tool for testers and developers.
Table of Contents
What Are DataProviders in TestNG?
DataProviders simplify data-driven testing, which runs test cases with multiple data sets. Instead of writing separate methods for each data set, you can pass data directly to test methods. This approach saves time, reduces redundancy, and ensures thorough testing. With DataProviders, you can validate that your application handles a range of inputs effectively.
Why Use DataProviders?
DataProviders excel in streamlining the testing process:
- They enable clean and efficient data-driven testing.
- You can test a method against multiple scenarios with minimal code duplication.
- Comprehensive tests ensure your application is reliable under diverse conditions.
Basics of DataProviders
Data-driven testing is key to modern software testing. DataProviders make it easier by allowing you to pass varied inputs to test methods.
Defining DataProviders
A DataProvider is a mechanism that feeds different sets of data into test methods. Here’s an example:
@DataProvider(name = "testData")
public Object[][] provideData() {
return new Object[][] { {"data1"}, {"data2"}, {"data3"} };
}
DataProviders vs. TestNG Parameters
Both DataProviders and parameters pass data to test methods, but they work differently.
- TestNG Parameters: Best for single-value inputs.
- DataProviders: Ideal for testing multiple input sets in one go.
Structuring DataProviders
DataProviders return a 2D object array, allowing you to test multiple parameters. The setup is straightforward and ensures your tests cover a range of scenarios.
For example, to test a login feature with multiple credentials:
@DataProvider(name = "loginData")
public Object[][] provideLoginData() {
return new Object[][] { {"user1", "pass1"}, {"user2", "pass2"} };
}
Here, each array represents a username and password pair for login tests. This method keeps your tests efficient, organized, and ready for diverse input scenarios.
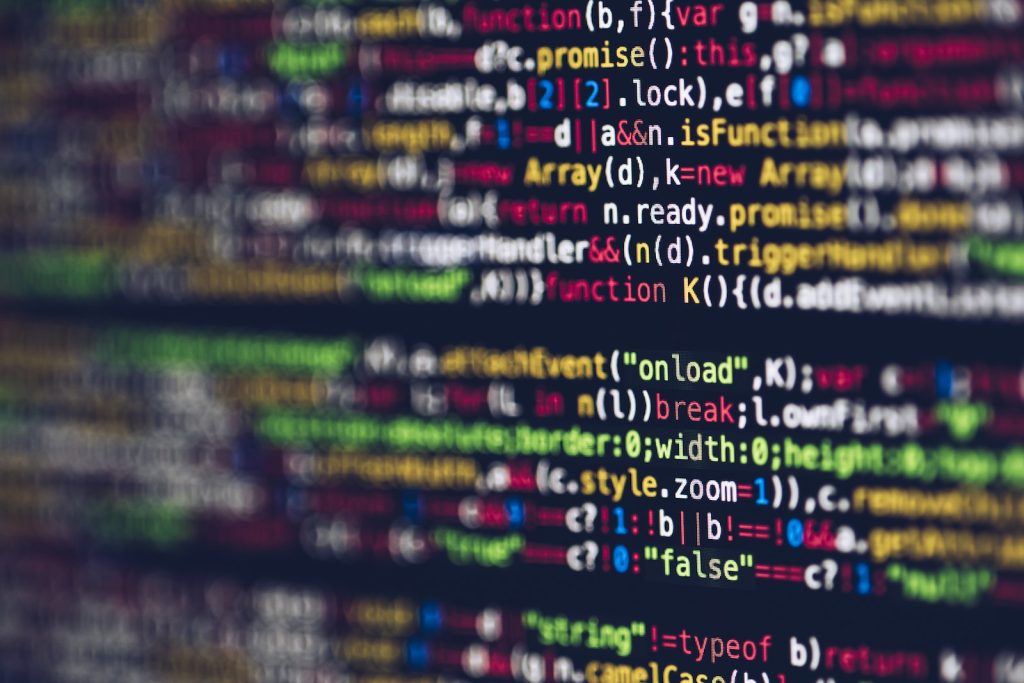
Crafting TestNG DataProviders Like a Pro
In TestNG, the @DataProvider
annotation acts as a key to unlock dynamic, flexible testing. Its true power comes from knowing how to use it effectively.
What Is the @DataProvider
Annotation?
The @DataProvider
annotation tells TestNG where to fetch test data. Think of it as pointing to a specific method for your test inputs.
@DataProvider(name = "sampleData")
public Object[][] fetchSampleData() {
return new Object[][] { {"dataA"}, {"dataB"} };
}
Here, the fetchSampleData
method provides data sets labeled as sampleData
.
Using DataProviders in Tests
Declaring and linking a DataProvider is simple. Once a method is annotated with @DataProvider
, you reference its name in your test method.
@Test(dataProvider = "sampleData")
public void sampleTestMethod(String data) {
System.out.println("Testing with: " + data);
}
This setup runs the sampleTestMethod
twice—once with dataA
and then with dataB
.
The Importance of Naming
Naming your DataProvider serves two purposes:
- Identification: A clear name helps you quickly identify the data source.
- Reference: The name connects your test method to the DataProvider.
But if you skip naming, TestNG automatically uses the method name as the default DataProvider name. This gives you the flexibility to either specify a name or let TestNG handle it.
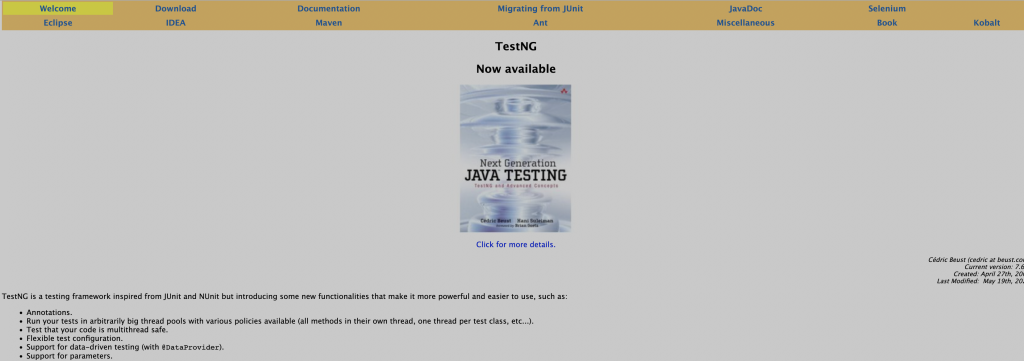
Implementing TestNG DataProviders in Real-World Scenarios
Now that we’ve covered the basics of @DataProvider
, it’s time to see it in action. Let’s explore how to apply DataProviders in real-world tests.
Step-by-Step: Building a DataProvider Test
1. Import Necessary TestNG Annotations
First, make sure to import the required TestNG classes:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
2. Create the DataProvider Method
Define the data sets your test will use:
@DataProvider(name = "loginCredentials")
public Object[][] provideLoginData() {
return new Object[][] { {"Alice", "password123"}, {"Bob", "passBob"} };
}
Here, we create a loginCredentials
DataProvider with two sets of username-password pairs.
3. Write the Test Method
Now, write the test method and link it to the DataProvider using its name:
@Test(dataProvider = "loginCredentials")
public void loginTest(String username, String password) {
System.out.println("Logging in with: " + username + " and " + password);
// Add your login test logic here
}
Each pair of credentials will be passed to the loginTest
method during execution.
4. Run the Test
Run the test through your IDE or command line. TestNG will execute the loginTest
method for each data set.
Deciphering the Output
The console will display:
Logging in with: Alice and password123
Logging in with: Bob and passBob
This output confirms that TestNG executed the test for every set of data, ensuring thorough coverage.
The Takeaway
DataProviders enable dynamic, data-driven testing by letting you reuse test logic with multiple inputs. This reduces redundancy, ensures comprehensive validation, and highlights potential issues across scenarios.
Using Inheritance to Share DataProviders
Inheritance lets you define DataProviders in one class and use them across others. Here’s an example:
Base Class with a DataProvider
public class BaseDataProviderClass {
@DataProvider(name = "sharedData")
public Object[][] provideSharedData() {
return new Object[][] { {"Data1"}, {"Data2"} };
}
}
Test Class Inheriting the DataProvider
public class TestClass extends BaseDataProviderClass {
@Test(dataProvider = "sharedData")
public void inheritedTest(String data) {
System.out.println("Testing with: " + data);
}
}
When you run the inheritedTest
method, TestNG will pull data from the provideSharedData
method in the base class.
Why Decouple DataProviders?
- Cleaner Code: Separating data from test logic improves readability.
- Reusability: A single DataProvider can be used across multiple tests.
- Easy Maintenance: Changes to data don’t affect test logic, making updates seamless.
Handling Multiple Parameters in TestNG DataProviders
Dealing with multiple parameters in testing can feel like juggling—it’s tricky, but with TestNG DataProviders, it’s a whole lot easier. Whether you’re testing logins, forms, or complex workflows, TestNG DataProviders let you handle multiple inputs without breaking a sweat.
Why Use DataProviders for Multi-Parameter Testing?
Think of it like this: every parameter in your test is an ingredient in a recipe. Missing one can ruin the whole dish. With TestNG DataProviders, you can test different combinations of parameters in a single method. For example, let’s say you’re working on a registration form. You can test usernames, passwords, and emails together without writing separate test methods.
Here’s how you can set up a DataProvider for multiple parameters:
@DataProvider(name = "registrationData")
public Object[][] provideRegistrationData() {
return new Object[][] {
{"Alice", "passAlice", "alice@email.com"},
{"Bob", "passBob", "bob@email.com"}
};
}
Writing a Test for Multiple Parameters
Once the DataProvider is ready, it’s time to put it to work:
@Test(dataProvider = "registrationData")
public void registrationTest(String username, String password, String email) {
System.out.println("Registering user: " + username);
System.out.println("With email: " + email);
// Your actual registration logic goes here
}
When you run this test, it will execute once for each set of data. This setup saves time and reduces repetitive code.
Understanding the Output
After running the test, you’ll see something like this in the console:
Registering user: Alice
With email: alice@email.com
Registering user: Bob
With email: bob@email.com
This output shows exactly how your tests handled each set of inputs. It’s clear and makes debugging easier if something goes wrong.
Integrating Method Parameters with TestNG DataProviders
If you want your DataProvider to be extra smart, you can make it respond to the test method that’s calling it. This trick, called integrating method parameters, lets you customize data for specific tests.
What Are Method Parameters in DataProviders?
Imagine your DataProvider as a vending machine. Depending on the button (method) you press, you get a different snack (data). TestNG lets you do exactly that by passing the method name to your DataProvider.
Here’s an example:
@DataProvider(name = "dynamicData")
public Object[][] provideData(Method m) {
if (m.getName().equals("testMethodA")) {
return new Object[][] { {"DataForA"} };
} else {
return new Object[][] { {"DataForB"} };
}
}
Setting Up the Tests
Now, use this dynamic DataProvider in your test methods:
@Test(dataProvider = "dynamicData")
public void testMethodA(String data) {
System.out.println("Testing Method A with: " + data);
}
@Test(dataProvider = "dynamicData")
public void testMethodB(String data) {
System.out.println("Testing Method B with: " + data);
}
When you run testMethodA
, it gets DataForA
. testMethodB
gets its own data, DataForB
.
Why This Approach Works
- Custom Data: Each test method gets exactly what it needs.
- Less Code: No need for separate DataProviders for every test.
- Easy Maintenance: If the data changes, you only update one place.
Wrapping It All Up
TestNG DataProviders are a game-changer for handling multiple parameters and customizing data for tests. They keep your code clean, make tests more efficient, and ensure you’re covering all the right scenarios. Whether you’re just getting started with TestNG or refining your testing strategy, mastering DataProviders is a skill you’ll use again and again.
Happy testing!